Install program & run for Trial Period for Try before you buy with AHK
Previous articles have discussed counter uses for a program and a license check to run. I’m interested in duration, as in, the software will run for 30 days then stop. So how to do?
On installing a program it has to go and get today’s date and secrete that somewhere. Then each subsequent time the program is run it needs to get the date NOW() and compare it with the start date, if its within bounds then it will allow program to run, if not, it won’t run the program.
So its really calculating basic subtraction but with dates.
Jack Dunnings forum post Function Calculating Timespan in Years, Months, and Days talks about some of the issues.
In AHK docs there are a couple of topics about time, and comparing time and there is an EnvAdd function that can add/subract time from date-time.
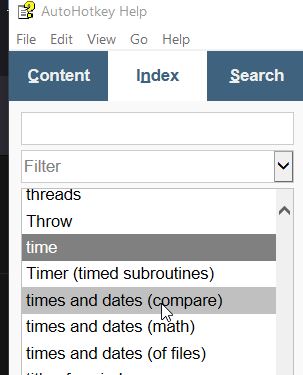

Jack also has this article The HowLongYearsMonthsDays.ahk Script Calculates Time Spans—This New DateCalc() Function Yields New Dates Based on Adding or Subtracting Years, Months, or Days which raises important issues about year and month variances, but if the program runs for 31 days or 29 its not a massive issue. So there is no need for a high degree of accuracy. If the EnvAdd function is the inbuilt AUTOHOTKEY function then I should give that a crack first.
Process
Get start date
So we need to first grab the time at install, put this somewhere in a readable file (.ini files key/value pairs are handy for this) and then do something to stop that file being overwritten.
A couple of things come to mind. 1/ Rename the file after putting date in it on first run of program, so 2nd time it runs, it looks for the original file and if not there then it does nothing, or 2/ have a counter in the .ini file set initially at 0, so first time it runs, it checks number, if <1 then write date, otherwise do nothing.
Calculate time period End date
So we now have original date, we now need to calculate an end date, be it 1, 5, 10, 30 days in the future.
This can be calculated and sent to the .ini file at the same time as start date is done, we only need to do it once inside the if <1 check above.
Get current Date/Time & compare with End date
So after that we just need to get current date and time and put it in a variable and compare that with the End date that we pull from the ini file.
If End Date – Current Date = ? Positive – run program, negative – trial time ended and you can choose to stop using program or purchase a License.
License install/request email.
This process is the same as per this article How to protect final program when distributing? License keys? where code is available.

Unix Epoch time
Unix time (UT) is just a single number, so you could calculate a date-time in the future and then set that number and keep on gathering current date time and converting it into Unix time and seeing if its less than the specified final number.
An interesting event happening in 2038 with 32 bit computers where the Binary date resets, read Y2k38 Epochalypse and video :
This may involve getting a UT function to convert date time into the correct format to do this.
I would like to use the lightest way possible as I want to minimise the code overhead on the program as it will be run every time the program is run.
A-NowUTC is also available in AutoHotKeys so that may be a better approach. You just have to find the number for your Duration – 1, 5, 10, 30 days etc & add it to A-NowUTC (Start) and then easily compare A-NowUTC(current) with A_NowUTC(Start)+Duration.
30 day duration is 60s x 60 min x 24 hrs x 30 days = 2592000 seconds
So 30 day license = A_NowUTC(Start)+ 2592000
Files
TimeTrial.ahk
/*
TimeTrial.ahk
This is a setup test for sharing files with others that will run for a specific TIME period then stop
The setup is planned to allow others to test the compiled code for X number of DAYS then it stops working
[email protected]
*/
;-----------------------Setup -----------------------------
#NoEnv
#SingleInstance, Force
SetWorkingDir %A_ScriptDir%
;-----------------------Include libraries -----------------------------
#Include %A_ScriptDir%\Notify.ahk
#Include %A_ScriptDir%\E13.ahk
;------------------Package ID ----------------------
PackageId = TimeTrial_v0.1_20211028
NgSplit=xNvRy
;-----------------------Files & Locations -----------------------------
; Trig = %A_AppData%\MSys\trigger.ini
Trig = trigger.ini
;------------------Prog ----------------------
;------------------Check # times program has run ----------------------
IniRead, run, %Trig%, Count, run
; if less than 1 then get start date and end trial time
if (run<1) ; this only runs once at the first run, its skipped all other times
{
EndTime = %A_NowUTC%
EndTime +=2592000 ;(30dayx24hoursx60minx60sec)=2592000
IniWrite, %A_NowUTC%, %Trig%, SDate, StDate ; write start date
IniWrite, %EndTime%, %Trig%, EDate, EndDate ; write end date
run+=1 ; increment number of times program run by 1
IniWrite, %run%, %Trig%, Count, run
}
;------------------Subroutine getting License info ----------------------
comp= %A_ComputerName%
user= %A_UserName%
Ip1=XXX.XXX.XX.XXX ;%A_IPAddress1%
Ip2= %A_IPAddress2%
Ip3= %A_IPAddress3%
Ip4= %A_IPAddress4%
sendx:= Rot13(comp)
underx:=Rot13(user)
IniWrite,%PackageId% , FileTemp.ini, p, PackageId
IniWrite,%sendx% , FileTemp.ini, c, cee
IniWrite, %underx%, FileTemp.ini, d, dee
IniWrite, %Ip1%, FileTemp.ini, Ips, Ip1
IniWrite, %Ip2%, FileTemp.ini, Ips, Ip2
IniWrite, %Ip3%, FileTemp.ini, Ips, Ip3
IniWrite, %uIp4%, FileTemp.ini, Ips, Ip4
IniRead, zed, %Trig%, serial, serialId
IniRead, why, %Trig%, other, B1
Timeframe =%underx%%NgSplit%%sendx%%why%
;********************************
;msgbox ,%zed% ** %Timeframe%
;********************************
;------------------Subroutine getting License info ----------------------
IniRead, EndT, %Trig%, EDate, EndDate ; get End date from file
If (EndT-A_NowUTC<0) ; Just a notification that you have some days left on trial
{
a=%A_NowUTC%
b=%EndT%
ToGo:=b-=a
DLeft:=Round(ToGo/=86400)
MsgBox, You have`n%DLeft% Days Left `nin your Trial period
}
If (EndT-A_NowUTC<0) ||(zed == Timeframe ) ; If current date less than end date OR if
{
/*
MsgBox, Hello world
*/
;------These for Notify popup class-----------------
Text:= "TimeTrial Started`n Enjoy"
Background=0x456789 ; a bluey color
Color=0xFFFFFF ; white;
Title = TIME TRIAL 30 DAYS ;This is my TITLE
TitleFont="Tahoma"
TitleColor= 0xFFFFFF ; white
TitleSize=20
Size=12 ;text height
Time= 3000 ;3000
Icon =145 ;297or145 tick,132 "x", 278 blue circle exclamation , 234 orange triangle exclamation; 220 no way,211 question in blue circle
IconSize =30
;------------------INITIATE INSTANCE OF NOTIFY ----------------------
Notify:=Notify()
;------------------NOTIFY POPUP STARTING THE PROGRAM ----------------------
Notify().AddWindow(Text,{Size:Size,Icon:Icon "," Ico,IconSize:IconSize,Title:Title,TitleColor:TitleColor,TitleFont:TitleFont,TitleSize:TitleSize,Background:Background,Color:Color,Time:Time}) ;Flash:1000,
sleep, 3000
ExitApp
}else{
MsgBox, Your time Trial Has expired`nHopefully you have found this program of use`nIf you wish to purchase the program`npress the REQUEST Key in the following Pop-up box`nTo enquire about purchasing a Licence
run, PC&UerInfoLicenceInstall.ahk ; PC&UerInfoLicenceInstall.exe
ExitApp
}
trigger.ini
[Count]
run=1
[SDate]
StDate =0211020000000
;20211028011507
;20211027202320
[EDate]
EndDate =20211030000000
[name]
nameId=1243
[serial]
serialId=qenxrxNvRyQRFXGBC-4X3PYRIpBvx7y
;serialId=av7x0x456789*QRFXGBC-VXTMQ*rixol
[other] ; this is 2nd part of key for License
B1=pBvx7y
PC&UerInfoLicenceInstall.ahk, LicenseRequest.ahk, FileTemp.ini, E13.ahk & Notify.ahk are all pretty much the same as in previous article
All files can be downloaded HERE.
Partial open Program- Freemium
Can use a cut down version of a program and then use a License to get access to more features. So simplified program runs but pay to unlock extra features.
Also you could give them the option of trialing all the features, including the locked off features, for a given period and if they like that then when it reverts to the basic features they have to purchase the license.
I think this is dependent on what your program is doing. It needs to be useful enough in its basic state and offer some sort of productivity as a standalone program but you lever far more efficiencies if you purchase the full program.
Some freemiums I’ve tried have been pretty useless in the cut down versions such that you wouldn’t bother with them, and others are useful enough in free plan not to bother purchasing the License for extra features.
I’m wondering what program I can test this out on. Maybe TimeCapture- Add feature that has clock that runs so that you can see what time duration has passed in program. So add a timer in the program that will run and a pop-up that you can select time for to notify a specific duration has passed.
You could also add an output method to structure data to PDF or add an expense part to the process.
Feedback Form
Maybe a feedback form at the end of the trial would be a handy thing. This may be something worth looking at. It could just be a link to a website or a Google Form!
A blank page that people could gve their views on how the software worked for them & their expectations of what they thought it would do and what they wanted it to do.
End comment
Its interesting exploring different licensing structures and how they would work with a program. I’ll have to develop and structure a program to test.
Maybe a feedback form at the end of the trial would be a handy thing. This may be something worth looking at. It could just be a link to a website or a Google Form!