AHK Barcode & Freezer Inventory
After plant watering/recording app, which I use daily I wanted to move onto the Freezer Inventory.
I have modified the Plant watering app for my beer brew recording, that is mainly typing in rather than barcode reading, but I inputted all the data pretty quickly, so now have a structured file with the data in it, a lot missing, but it’s a start.
The Freezer inventory app is a bit more challenging. I’m going to use the template of the Plant watering app, as that has 1/ A GUI interface with Text to Speech & 2/ It records data to a file.
For the first try I want to keep this simple and use a Text file for records, I do not want to move to an Excel/GSheets spreadsheets with code lookups using VLookup at this point. Although there is this AHK article Please Help! Using of VLOOKUP that I may explore later.
Items that I think I need to make this happen:
- popup to add items
- Popup to search for items and return
- Popup to add to/take away from current count
I also want to use the Text File Library that has a lot of useful functions that I can use, and I’ll use # include tf.ahk to work with some of the following items in the library:
- TF _Find
- TF_Replace
- TF_ReplaceInLines
- TF_CountLines
Coding
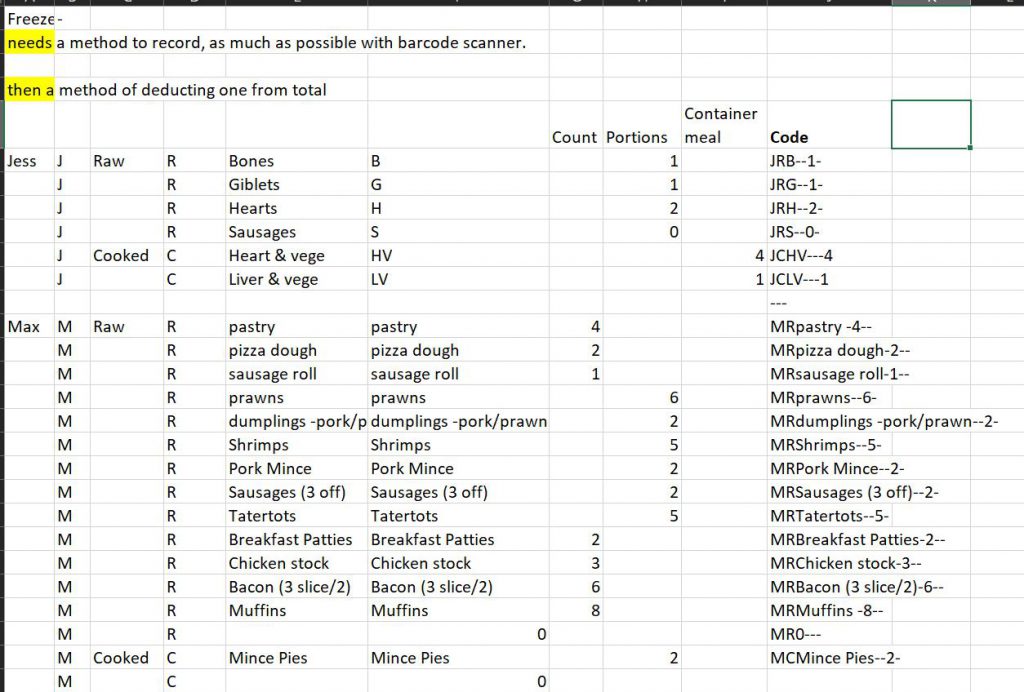
The first issue was getting an abbreviation code method that worked. Started witj Letters as abbreviation – Who For (dog or me, J or M), Raw or Cooked (R,C), then abbreviations for what they were, pretty simple for the dog , only one or 2 letters, for me a bit more elaborate.

So, for just the naming I need to do some abbreviations on the more complex things in the freezer.
The 2nd issue is count. There are individual items , eg Bones, and there are portions, such as a portion of chicken hearts or Giblets, or there are containers with cooked food in a package.
So you can either reduce everything to a single unit in some complex calculation method, or work with at least 3 different quantity units.
In some ways, I should start off with a single count unit, just to get a minimum prototype working, maybe using count, then extend to different units. Why is that? See next heading
Populating file
The next thing I need to do is populate a file to start with. So best method for this is to have a pop-up GUI that will allow me to easily add things to the existing file. This is simple, the basis of the plant watering app. Just scan in a new code and a number (Quantity).
Reading & editing file
This is the biggy. You have to put in a Key search, so it needs to loop through all the lines in the file to find the one that you want.
This would be the Code of the item. It would then look up the code and return the quantity. At this point you know how many of the “code” thingy are available.
If you use one or 2 of them, you need to be able to deduct this from the current amount in the file, so you need to edit the file for the update.
If you add to the file more of a specific “code” then you need to be able to modify the file again as well.
Date/Time will be your friend here when you change records, you need to overwrite previous date/times.
The thinking is that you will only have one line pertaining for a single code item and you’ll modify it every time you do some action such as remove or add more of that specific item and you modify date/time to identify last time that was updated.
You could create a backup file with the datetime appended to it, so have stored lots of files with previous data, one method of having a record of all the transactions.
I don’t want to have each transaction as another line in the file otherwise the search function will take longer and longer as the file gets bigger after many transactions. I’m only interested in what’s in the freezer at this point, so a simple lean file will be quick to loop through.
Actual working code
So after all the exploring and trying things, I ended up resolving issues in the following ways:
Code, description, count & file structure
Code. As QR codes need to be simple if they are only going to ne 8mm high, I decided on 5 digits. Codes can give a hint of what they mean like jbone (bones for Jess) or jht&v (jess Heart & vege), so you have a bit of an indication of what they mean.
Description. As the code is “coded” you need a description to explain what the item is, and this can be verbose :
- jbone– Jess beef bones, number
- jht&v -Jess Heart & vege cooked meal container
As this can vary in length, I put it at the end of the row, therefore it can grow as long as you want without affecting file structure (see below)
Count. Now there will normally less than 10 items in batches, but to allow for some flexibility if you allow for 2 digits you can get up to 99 of anything. So I use 01, 02,……09,10, 11 …..35, so I need a leading zero on the end of numbers to 9.
As we are using a text file, this is not a problem, as txt files have no issue with a leading zero, whereas , if you brought the file into a spreadsheet it will get rid of the leading zero. As part of my coding learning I decided that I wanted to stick with a simple .txt file format.
The leading zero is important, in that it allows you to have a simple file structure with different count types, such as number, portion & container.
Fle structure. With all the items having the same format, Code, Number, Portion, Container, Date, Description all the items apart from the last (Description) have a standard length.
jcdrm,08,00,00,26-02-2021,J chicken Drumsticks raw
jgibl,00,02,00,26-02-2021,J Giblets raw
mshmp,00,05,00,26-02-2021,m shrimp 1kg bag raw 200g/portion
mprwn,00,05,00,26-02-2021,m prawn 1kg bag 200g /portion
mpies,02,00,00,26-02-2021,m pies mince
mdump,00,02,00,26-02-2021,m dumplings portions (8 to 10)
mroti,10,00,00,26-02-2021,m roti chenai
mbacn,00,06,00,26-02-2021,m bacon 4 slices per portion
mmuff,08,00,00,26-02-2021,m muffins english breakfast count
msw&s,00,00,01,26-02-2021,m sweet & sour pork meal
mpkms,00,01,00,26-02-2021,m pork mince portion 500g
mtots,00,05,00,26-02-2021,m tatertots bag 750g
mbpat,02,00,00,26-02-2021,m beef burger patties
mfrnk,00,05,00,26-02-2021,m frankfurters portion
When you do a search for a code, it returns a row of data, which is basically a long string.
Although we used variables to put this information into the file in a specific method, once in the text file it is just a string, with deliminators between them.
So on retrival of a searched code you get a row of data you can use SubSt() to assign each part a new variable and can therefore do string manipulation on those substrings.
So once the specific string is broken down into variables you can then update those variables and pus them back into the file, updated to new condition.
Process
Although I had a script setup for submitting new items to the file, I actually found it easier just to use Google Sheets and a concatenation process to set it all up, it went a lot quicker.
I’ve started using if/else statements to simplify the input areas (eg if portion is not 00 then only show portion (for editing) and auto-populte number, container with 00. So this speeds up the process of setup.
I was miserly with label tape and only printed the QR code, the rest I did by hand
Text file line modify/line delete.
I used the tf.ahk library (mentioned above) and spent a lot of time with it with minimal success, then found a simple function online that would delete a line in a file by looping though all the lines and re-writing them apart from the line that you want to delete.
Barcode scanner Enter command
When doing search, there is a pop-up inputbox that you need to put in code , then press enter. Also the Gui for altering the string returned needed a press OK at the bottom, another Enter.
With the plants input for scanner you have Data + Tab or Data+ Carriage return (enter). I wanted just Enter on its own and couldn’t find it in the codebook, so I thought I’d try AHK Send, {Enter} and that seemed to work. So I didn’t have to reconfigure settings on the scanner.
So, I haven’t refined the code yet to see if I can remove the enter buttons but just scan a Send, {Enter} QR code and that works.
For the moment its another step, but the process is robust. I can always refine the code later. Great to know that there is a hack for that without reprogramming scanner.
End comment
An interesting project fro learning about file /string manipulation. I have a working process, so will use and see if its effective and usable.
I’m glad I used the Text file instead of spreadsheet, evilC had some code on using bar scanner with excel, and it’s a bit complex and you have to fire up excel and a specific file to use.
Code for download
0-InventoryEdit.ahk , 0-InventoryNew.ahk, 0-FreezerInv.csv