Building an API in Node
This is a cover block I’m trying out
I’m going to try and follow this tutorial, some of the early ones are stuff I’ve done before, but the reference to the npm video was interesting. Also to the npm site for looking at packages.
Two other resources I just want to highlight. 1/ Daniel Shiffman website and 2/ npm website for packages.
Objectives
First objectives with API
After playing around with Node.js I’m starting to get the feel of it and what it does, now I need to reconfirm my objectives. I want to:
- scrape a site and get data from a table,
- add the data to a JSON file, this is not necessarily the case actually, I just need, in the terms he uses in the videos “persistence” where I can store the data, (and I think at the end of this it will be a database table and making calls) so I need to get some data ,
- store some data
- serve that data up
2nd Objective– hosting the node server
Next I need to host the Note server instance, so I think this will be another post, and I think the first part is doing it on a Horuku site , but I have a feeling I’ll need to go via Github.
I want to be able to have the server running continuously so I can make requests to it at anytime from anywhere.
The building an API in Node tutorial
tut 1 & 2 ok, tut 8.2 have initial setup running, all basic, no problem. Now Routes, I thought this was interesting for linkages and API calls , especially with queries to aPI’s. So starting that tutorial now:
So using :
app.use(express.static('website'));
app.get ('/flower', sendFlower);
function sendFlower(request, response){
response.send("I think flowers are nice too");
}
you can go to the server and : http://localhost:3500/flower add the /flower on the end it feeds back information without the HTML page doing anything. So this is calling a function and rendering the result directly to the page.
This is something different and I need to get my head around this. I didn’t know you could do that!
So the next stage is : app.get (‘/search/:flower’, sendFlower);
This means the ‘/search/ is the route annd the “ :” is something that the user wil give which will be put in thr REQUEST. So in http://localhost:3500/search/poppy, I added poppy, so that came out in the page, not written on the index.html page but rendered directly from JavaScript.
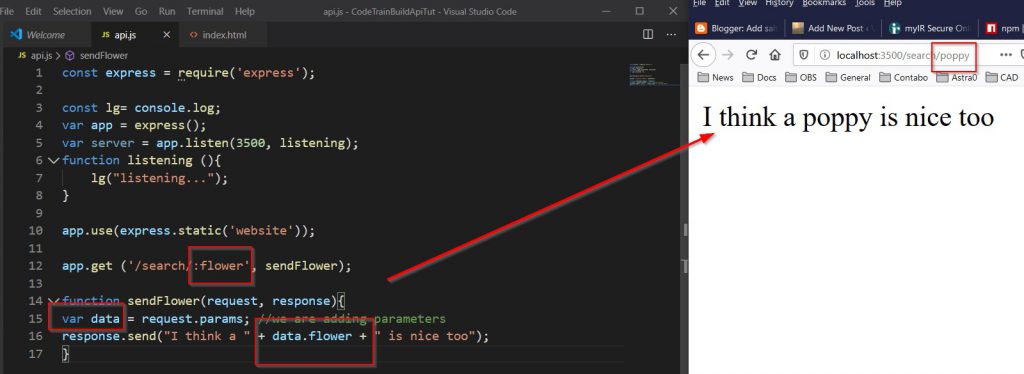
So the final tut show iteration where if you put in a flower, then number, it will iterate through and repeat the text the number of times you’ve put it in.
const express = require('express');
const lg= console.log;
var app = express();
var server = app.listen(3500, listening);
function listening (){
lg("listening...");
}
app.use(express.static('website'));
app.get ('/search/:flower/:num', sendFlower);
function sendFlower(request, response){
var data = request.params; //we are adding parameters
var num = data.num;
var reply= "";
for (var i=0; i<num; i++){
reply += "I think a " + data.flower + " is nice too <br>";
}
response.send(reply);
}

So, in this tutorial we have defind a route and also added parameters to the route with keywords. The route /Search, and the keywords /:flower (you specify) /:number (you specify)
Tut 4 – sentiment analysis
This is about adding some persistance using json data in a sentiment analyser. So follwoing video wen well, so can now get all the DB, add to the DB & search in the DB. But no persistence if you restart the server, as the file is not saved, so added values are not saved. I’m putting code below as it alters over the tutorials, so its good to have an indication of what happens at each step, easier to go back and check code.
So 3 routes created, “/all”, “/add” & “/search”
const express = require('express');
const lg= console.log;
var words={
"happy" : 2,
"joy": 3,
"euphoria":8,
"gloom": -2,
"melancholy": -1,
"despondancy":-4
}
var app = express();
var server = app.listen(3500, listening);
function listening (){
lg("listening...");
}
app.use(express.static('website'));
app.get ('/add/:word/:score?', addWord);// adding ? to last will make it optional
function addWord(request, response){
var data = request.params; //we are adding parameters
var word = data.word;
var score =Number(data.score);
var reply;
if(!score){
reply={
msg:"Score is required."
}
} else {
words[word] = score;
reply={
msg:"Thank you for your word."
}
}
response.send(reply);
}
app.get('/all', sendAll);
function sendAll(request, response){
response.send(words);// words is structured as JSON so express will send it out as JSOn
}
app.get ('/search/:word/', searchWord);
function searchWord(request, response){
var word = request.params.word;
var reply;
if(words[word]){
reply={
status: "found",
word: word,
score: words[word]
}
}else {
reply = {
status: "NOT found",
word: word
}
}
response.send(reply);
}
T5 Saving data to JSON file
This is creating a JSON file as store of data for persistance and then loading file, syncronously, so data loads first before it goes to next step, then can add to the file, but write asyncronously (otherwise it re-loads all the file again, which we do not want to happen each time.
const express = require('express');
const fs = require('fs');
const lg= console.log;
var app = express();
var server = app.listen(3500, listening);
function listening (){
lg("listening...");
}
var data1= fs.readFileSync('words.json');//readFileSync means that the data all has to be loaded before it goes to the next step.GOOD
var words=JSON.parse(data1);
lg (words);
app.use(express.static('website'));
app.get ('/add/:word/:score?', addWord);// adding ? to last will make it optional
function addWord(request, response){
var data = request.params; //we are adding parameters
var word = data.word;
var score =Number(data.score);
//var reply;
if(!score){
var reply1={
msg:"Score is required."
}
response.send(reply1);
} else {
words[word] = score;
var data = JSON.stringify(words, null, 2);
fs.writeFile('words.json',data, finished);// this is not writeFileSync ans we dont want to re-upload file each time, so just writeFile
function finished(err){
lg('word added');
var reply2={
word: word,
score: score,
status : "success. Thank you for your word."
}
response.send(reply2);
}
}
app.get('/all', sendAll);
function sendAll(request, response){
response.send(words);// words is structured as JSON so express will send it out as JSOn
}
app.get ('/search/:word/', searchWord);
function searchWord(request, response){
var word = request.params.word;
var reply;
if(words[word]){
reply={
status: "found",
word: word,
score: words[word]
}
}else {
reply = {
status: "NOT found",
word: word
}
}
response.send(reply);
}
}
T6 Showing Clientside on page & T7 doing sentiment analysis
I just watched these videos as I’m not particularly interested in this part except for the POST and COR’s parts in video T7. He went through those pretty fast, so I’ll need a project to look at to go back and use. He also did a lot with the P5.js package which I haven’t used so I may have to go and look into that later.
End comment
For the moment I wonder if I can add HTML into the information coming back from get request so that it renders in the browser (it didn’t , although I did use linebreak somewhere and that worked.
P5.js
Also I may have to go back and do a bit with the P5.js modules. Actually that looks quite graphical, pretty but you can draw elements with it so may be worth exploring for something like responsive plans
Objectives form Tutorials. Good but I still need a lot more from other sources.
From First objectives with API on the list I think I have an ideaon how to use put data into a JSON file and how to access it, server side, (items 2/3) so I need to konw how to append to a file. But first I need to get the NodeJS server running on Horuku or something similar, I need a live source to play with.
From previous post I have got information from tables, now I have to combine that with what this series has taught me to try and build a JSON file and append the data.
Later I’ll need to render it client side, which will mean calling that data.